This documentation includes the steps for integrating a payment gateway using the Inline JavaScript (JS) method. The provided code sample includes an HTML document with an embedded script to trigger a checkout modal. This method allows customers to complete the payment process in a popup modal, ensuring a seamless experience without redirecting to another page.
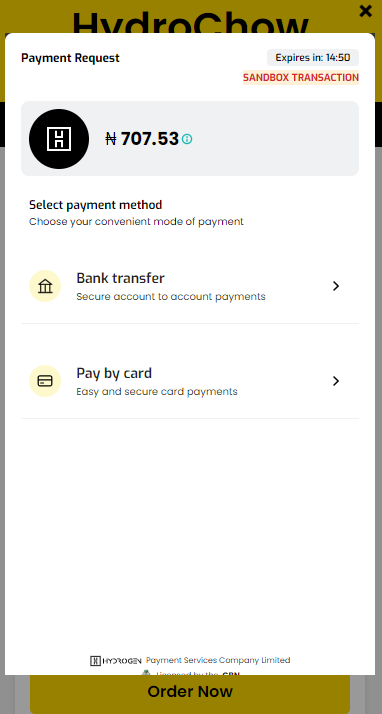
Hydrogen Popup Checkout
Request Parameters
Name | Description | Required |
---|---|---|
amount | The amount to be charged for the transaction. | Yes |
The customer's email address. | Yes | |
currency | The currency in which the transaction is to be processed (e.g., "NGN"). | Yes |
description | A brief description of the transaction. | Yes |
meta | Additional metadata or information related to the transaction. | Yes |
Integration Steps
1. Include the Required Scripts
Include the necessary script in the **<head>**
section of your HTML document.
<script src="https://js.hydrogenpay.com/inline.js" module></script>
2. Create a Checkout Button
Add a button to your HTML page that will trigger the checkout modal when clicked. This button will call the openDialogModal() function.
<button id="myBtn" onclick="openDialogModal()">Checkout</button>
3. Include Payment Information
Set up a JavaScript object (obj) with the necessary parameters for the payment transaction. This object will be used to initiate the payment link through an API.
let obj = {
amount: 10,
email: "[email protected]",
customerName: "Test User",
currency: "NGN",
description: "test desc",
meta: "test meta",
isAPI: true,
};
4. Authorization Token
Copy an authorization token (token) from the Hydrogenpay dashboard based on your testing or production requirements.
// For Sandbox
let token = "E2E411B102072296C73F76339497FB8529FF552F0D6817E0F3B46A243961CA21";
5. Payment Gateway Function
Implement the openDialogModal function, which calls the handlePgData function from the external script with the defined payment information and token.
async function openDialogModal() {
let res = handlePgData(obj, token);
console.log("return transaction ref", await res);
}
Following these steps will allow you to integrate the provided code sample into your web application, enabling a seamless payment gateway experience with an inline JavaScript popup.